Table of Contents
Write and read data
In Src/main.c add
#include “stm32f7xx_hal.h”
#include “stm32f7xx_hal_uart.h”
#include “stm32f7xx_hal_sdram.h”
#include “stm32f7xx_hal_ltdc.h”
#include “stm32f7xx_hal_qspi.h”
#include “stm32746g_discovery.h”
#include “stm32746g_discovery_lcd.h”
#include “stm32746g_discovery_qspi.h”
#include “stm32746g_discovery_sdram.h”
#include “stm32f7xx_ll_fmc.h”
#define BUFFER_SIZE 60
#define WRITE_READ_ADDR ((uint32_t)0x0000)
void SystemClock_Config(void);
void Error_Handler(void);
static void MX_GPIO_Init(void);
int main(void)
{
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
BSP_LED_Init(LED1);
uint8_t WriteBuffer[BUFFER_SIZE] = “Hello world ! This is a test of QuadSpi!”;
uint8_t ReadBuffer[BUFFER_SIZE];
BSP_LCD_Init();
BSP_LCD_LayerDefaultInit(0, LCD_FB_START_ADDRESS);
BSP_LCD_LayerDefaultInit(1, LCD_FB_START_ADDRESS+(BSP_LCD_GetXSize()*BSP_LCD_GetYSize()*4));
BSP_LCD_DisplayOn();
BSP_LCD_SelectLayer(0);
BSP_LCD_Clear(LCD_COLOR_BLACK);
BSP_LCD_SelectLayer(1);
BSP_LCD_Clear(LCD_COLOR_BLACK);
BSP_LCD_SetFont(&LCD_DEFAULT_FONT);
BSP_LCD_SetBackColor(LCD_COLOR_WHITE);
BSP_LCD_SetTextColor(LCD_COLOR_DARKBLUE);
BSP_LCD_SetBackColor(LCD_COLOR_WHITE);
BSP_QSPI_Init();
QSPI_Info pQSPI_Info;
BSP_QSPI_GetInfo(&pQSPI_Info);
if ((pQSPI_Info.FlashSize != N25Q128A_FLASH_SIZE) ||
(pQSPI_Info.EraseSectorSize != N25Q128A_SUBSECTOR_SIZE) ||
(pQSPI_Info.ProgPageSize != N25Q128A_PAGE_SIZE) ||
(pQSPI_Info.EraseSectorsNumber != N25Q128A_SUBSECTOR_SIZE) ||
(pQSPI_Info.ProgPagesNumber != N25Q128A_SECTOR_SIZE))
{
Error_Handler();
}
if (BSP_QSPI_Erase_Block(WRITE_READ_ADDR) != QSPI_OK)
{
Error_Handler();
}
if (BSP_QSPI_Write(WriteBuffer, WRITE_READ_ADDR, 60) != QSPI_OK)
{
Error_Handler();
}
if (BSP_QSPI_Read(ReadBuffer, WRITE_READ_ADDR, 13) != QSPI_OK)
{
Error_Handler();
}
BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)ReadBuffer, CENTER_MODE);
if (BSP_QSPI_Read(ReadBuffer, WRITE_READ_ADDR+13, 30) != QSPI_OK)
{
Error_Handler();
}
BSP_LCD_DisplayStringAt(0, LINE(5), (uint8_t *)ReadBuffer, CENTER_MODE);
while (1)
{
HAL_GPIO_WritePin(GPIOI, GPIO_PIN_1,1); HAL_Delay(1000);
HAL_GPIO_WritePin(GPIOI, GPIO_PIN_1,0); HAL_Delay(1000);
}
}
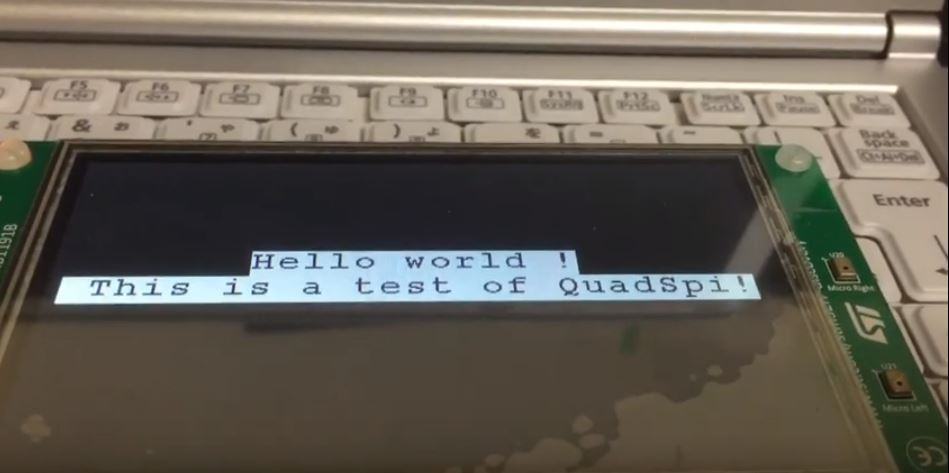