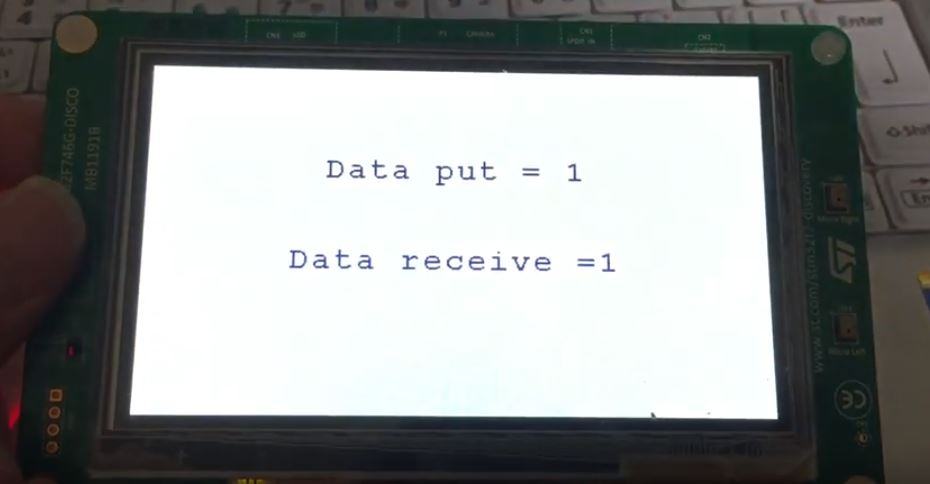
Table of Contents
Stm32f7-disco board used.
myTask02 --> Receive data
myTask03 -->Blink Led
1. Pin out setting
2. Free RTOS configuration
3. Source generation and edit code
In Src/main.c add
#include “stm32f7xx_hal.h”
#include “stm32f7xx_hal.h”
#include “stm32f7xx_hal_uart.h”
#include “stm32f7xx_hal_sdram.h”
#include “stm32f7xx_hal_ltdc.h”
#include “stm32746g_discovery.h”
#include “stm32746g_discovery_lcd.h”
#include “stm32746g_discovery_sdram.h”
#include “stm32f7xx_ll_fmc.h”
#include “cmsis_os.h”
osThreadId defaultTaskHandle;
osThreadId myTask02Handle;
osThreadId myTask03Handle;
osMessageQId myQueue01Handle;
void SystemClock_Config(void);
void Error_Handler(void);
static void MX_GPIO_Init(void);
void StartDefaultTask(void const * argument);
void StartTask02(void const * argument);
void StartTask03(void const * argument);
int main(void)
{
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
BSP_LED_Init(LED1);
BSP_LCD_Init();
BSP_LCD_LayerDefaultInit(0, LCD_FB_START_ADDRESS);
BSP_LCD_LayerDefaultInit(1, LCD_FB_START_ADDRESS+(BSP_LCD_GetXSize()*BSP_LCD_GetYSize()*4));
BSP_LCD_DisplayOn();
BSP_LCD_SelectLayer(0);
BSP_LCD_Clear(LCD_COLOR_BLACK);
BSP_LCD_SelectLayer(1);
BSP_LCD_Clear(LCD_COLOR_BLACK);
BSP_LCD_SetFont(&LCD_DEFAULT_FONT);
BSP_LCD_SetBackColor(LCD_COLOR_WHITE);
BSP_LCD_SetTextColor(LCD_COLOR_DARKBLUE);
BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)”Start_Free_RTOS”, CENTER_MODE);
HAL_Delay(1000);
osThreadDef(defaultTask, StartDefaultTask, osPriorityNormal, 0, 128);
defaultTaskHandle = osThreadCreate(osThread(defaultTask), NULL);
osThreadDef(myTask02, StartTask02, osPriorityIdle, 0, 128);
myTask02Handle = osThreadCreate(osThread(myTask02), NULL);
osThreadDef(myTask03, StartTask03, osPriorityIdle, 0, 128);
myTask03Handle = osThreadCreate(osThread(myTask03), NULL);
osMessageQDef(myQueue01, 16, uint16_t);
myQueue01Handle = osMessageCreate(osMessageQ(myQueue01), NULL);
osKernelStart();
while (1)
{
HAL_Delay(500);
}
}
————————————————————————————————
/*Receive data*/
void StartDefaultTask(void const * argument)
{
osEvent evt;
for(;;)
{
char buffer[15];
evt = osMessageGet(myQueue01Handle,1000);
BSP_LCD_Clear(LCD_COLOR_WHITE);
if (evt.status == osEventMessage) {
sprintf(buffer,”Data receive =%d”,(int)evt.value.p );
BSP_LCD_DisplayStringAt(0, LINE(6), (uint8_t *)buffer, CENTER_MODE);
} else {
BSP_LCD_DisplayStringAt(0, LINE(6), (uint8_t *)”Failed”, CENTER_MODE);
}
}
}
/*Send data*/
void StartTask02(void const * argument)
{
uint16_t i=0;
char buffer_1[15];
for(;;)
{
i++;
sprintf(buffer_1,”Data put = %d”,i );
osMessagePut(myQueue01Handle, (uint16_t)i, 500);
BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)buffer_1, CENTER_MODE);
osDelay(1000);
}
}
/*Blink led*/
void StartTask03(void const * argument)
{
for(;;)
{
BSP_LED_On(LED1);
HAL_Delay(500);
BSP_LED_Off(LED1);
HAL_Delay(500);
}
}
Video Stm32cubemx Free RTOS Exmaple