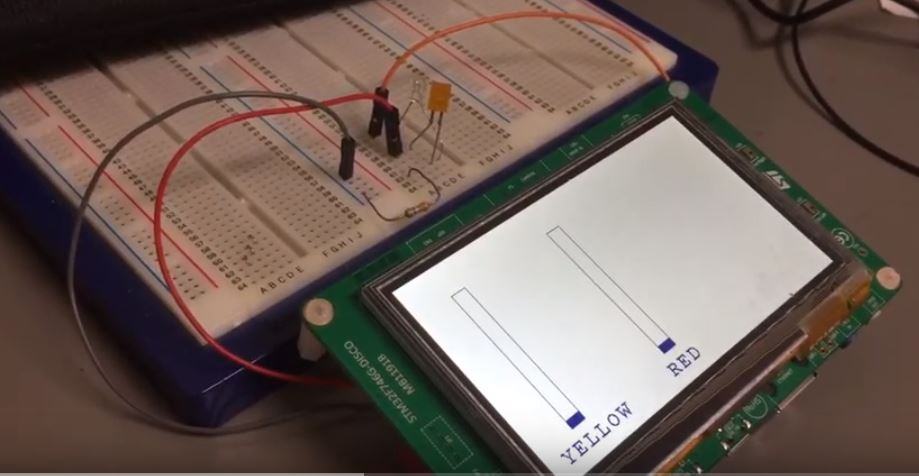
Table of Contents
Connect LED_1 to PA_8 and LED_2 to PA_15 pin.
After generate the code add this code to Src/main.c:
#include “stm32f7xx_hal.h”
#include “stm32f7xx_hal_uart.h”
#include “stm32f7xx_hal_sdram.h”
#include “stm32f7xx_hal_ltdc.h”
#include “stm32746g_discovery.h”
#include “stm32746g_discovery_ts.h”
#include “stm32746g_discovery_lcd.h”
#include “stm32746g_discovery_sdram.h”
#include “stm32f7xx_ll_fmc.h”
#include “stdio.h”
#include <stdbool.h>
/* USER CODE BEGIN Includes */
/* USER CODE END Includes */
/* Private variables ———————————————————*/
TIM_HandleTypeDef htim1;
TIM_HandleTypeDef htim2;
/* USER CODE BEGIN PV */
/* Private variables ———————————————————*/
/* USER CODE END PV */
/* Private function prototypes ———————————————–*/
void SystemClock_Config(void);
void Error_Handler(void);
static void MX_GPIO_Init(void);
static void MX_TIM1_Init(void);
static void MX_TIM2_Init(void);
void HAL_TIM_MspPostInit(TIM_HandleTypeDef *htim);
TS_StateTypeDef TS_State;
void led1(float duty){
TIM1->CCR1=(int)(duty*65535.0);
}
void led2(float duty){
TIM2->CCR1=(int)(duty*65535.0);
}
int main(void)
{
HAL_Init();
/* Configure the system clock */
SystemClock_Config();
/* Initialize all configured peripherals */
MX_GPIO_Init();
MX_TIM1_Init();
MX_TIM2_Init();
HAL_TIM_PWM_Start(&htim1,TIM_CHANNEL_1);
HAL_TIM_PWM_Start(&htim2,TIM_CHANNEL_1);
BSP_LCD_Init();
BSP_LCD_LayerDefaultInit(0, LCD_FB_START_ADDRESS);
BSP_LCD_LayerDefaultInit(1, LCD_FB_START_ADDRESS+(BSP_LCD_GetXSize()*BSP_LCD_GetYSize()*4));
BSP_LCD_DisplayOn();
BSP_LCD_SelectLayer(0);
BSP_LCD_Clear(LCD_COLOR_BLACK);
BSP_LCD_SelectLayer(1);
BSP_LCD_Clear(LCD_COLOR_BLACK);
BSP_LCD_SetFont(&LCD_DEFAULT_FONT);
BSP_LCD_SetBackColor(LCD_COLOR_WHITE);
BSP_LCD_SetTextColor(LCD_COLOR_DARKBLUE);
BSP_LCD_SetBackColor(LCD_COLOR_WHITE);
if( BSP_TS_Init(BSP_LCD_GetXSize(), BSP_LCD_GetYSize())!=TS_OK){
Error_Handler();
}
bool state_init=false;
char text[15];
int count=0;
uint16_t x[2],y[2],last_y[2];
uint16_t get_y,pre_y=0;
x[0]=50;
x[1]=200;
y[0]=220;
y[1]=220;
BSP_LCD_Clear(LCD_COLOR_WHITE);
BSP_LCD_FillRect(x[0],y[0],20,10);
BSP_LCD_FillRect(x[1],y[1],20,10);
BSP_LCD_DrawRect(x[0],40,20,190);
BSP_LCD_DrawRect(x[1],40,20,190);
BSP_LCD_DisplayStringAt(0, LINE(10), (uint8_t *)” YELLOW RED”, LEFT_MODE);
while (1)
{
BSP_TS_GetState(&TS_State);
led1(((float)(220-y[0])/180.0));
led2(((float)(220-y[1])/180.0));
count++;
if (TS_State.touchDetected) {
if(count>20){
BSP_LCD_Clear(LCD_COLOR_WHITE);
BSP_LCD_FillRect(x[0],y[0],20,10);
BSP_LCD_FillRect(x[1],y[1],20,10);
BSP_LCD_DrawRect(x[0],40,20,190);
BSP_LCD_DrawRect(x[1],40,20,190);
BSP_LCD_DisplayStringAt(0, LINE(10), (uint8_t *)” YELLOW RED”, LEFT_MODE);
count=0;
}
get_y = TS_State.touchY[0];
if(state_init==false){
state_init=true;
pre_y=get_y;
}
int16_t d_y=get_y-pre_y;
pre_y=get_y;
if(state_init==true){
memset(text,”,sizeof(text));
if( TS_State.touchX[0]>x[0]-30&&TS_State.touchX[0]<x[0]+50){
if(d_y>1){
y[0]+=2;
last_y[0]=y[0];
}
else if(d_y<-1){
y[0]-=2;
last_y[0]=y[0];
}
else if (d_y>=-1||d_y<=1){
y[0]=last_y[0];
}
if(y[0]<=40){
y[0]=40;
}
else if(y[0]>220){
y[0]=220;
}
}
if( TS_State.touchX[0]>x[1]-50&&TS_State.touchX[0]<x[1]+50){
if(d_y>1){
y[1]+=2;
last_y[1]=y[1];
}
else if(d_y<-1){
y[1]-=2;
last_y[1]=y[1];
}
else if (d_y>=-1||d_y<=1){
y[1]=last_y[1];
}
if(y[1]<=40){
y[1]=40;
}
else if(y[1]>220){
y[1]=220;
}
}
}
}
else{
state_init=false;
}
}
}